Infrastructure as a Code
Infrastructure as code is the process of automating your infrastructure (networks, virtual machines, load balancers, and connection topology) provisioning. It uses a descriptive coding language and versioning system that is like what is used for source code. Idempotence is a principle of Infrastructure as Code. Azure provides native support for IaC via the Azure Resource Manager. Teams can define declarative templates that specify the infrastructure required to deploy their solutions.
Azure Resource Manager template
Azure Resource Manager is a service and ARM Templates are a type of Infrastructure as Code. ARM templates are files that represent Azure resources. You write them in a special JSON format. They define the objects you want, their types, names, and properties in a JSON file. The ARM template syntax contains functions and methods to perform complex operations. They allow you to define your Azure Infrastructure using code in a declarative way. Rather than saying how you want things to be created (e.g., Azure CLI/PowerShell), you specify what you want to be created. Azure then ‘makes it happen’.
Introduction to Azure Bicep
The bicep is a “Domain-Specific Language for deploying Azure resources declaratively”. Probably the easiest way to think of it is that it’s simply ARM templates with a much nicer syntax. Bicep code is transpile to ARM templates. It certainly achieves the (not difficult) goal of being less verbose and easier for a human to read and write than an ARM template.
Bicep embraces modularity. You can create a Bicep file that defines your preferred way of configuring any resource (storage account, virtual machine). And then another Bicep template can include that definition, only needing to override parameters it wants to change. This means that over time, as you use Bicep more, you should find you can reuse pre-existing templates rather than needing to build everything up from scratch every time.
How to install Bicep tools
To create Bicep files, you need a good Bicep editor (I am using Visual Studio Code)
Let’s write our first Azure Bicep template
Parameters
Each parameter needs a name and type. A parameter can’t have the same name as a variable, resource, output, or other parameters in the same scope. By providing different values for parameters, you can reuse a Bicep file for different environments.
Allowed values
You can define allowed values for a parameter. You provide the allowed values in an array. The deployment fails during validation if a value is passed in for the parameter that isn’t one of the allowed values.
Length constraints
If we are not passing minimum length characters then it will throw the below error,
Default value
You can specify a default value for a parameter. The default value is used when a value isn’t provided during deployment. To specify a default value along with other properties for the parameter, use the following syntax.
Description
To help users understand the value to provide, add a description to the parameter. When deploying the template through the portal, the description’s text is automatically used as a tip for that parameter. Only add a description when the text provides more information than can be inferred from the parameter name.
To deploy a resource through a Bicep file, you add a resource declaration by using the resource keyword.
Set resource type and version
When adding a resource to your Bicep file, start by setting the resource type and API version. These values determine the other properties that are available for the resource.
The following example shows how to set the resource type and API version for a storage account. The example doesn’t show the full resource declaration.
You set a symbolic name for the resource. In the preceding example, the symbolic name is pip. You can use any value for the symbolic name, but it can’t be the same as another resource, parameter, or variable in the Bicep file. The symbolic name isn’t the same as the resource name. You use the symbolic name to reference the resource in other parts of your Bicep file.
Set resource name
Each resource has a name. When setting the resource name, pay attention to the rules and restrictions for resource names.
Set location
Many resources require a location. You can determine if the resource needs a location either through intelligence or template reference. The following example adds a location parameter.
Set resource-specific properties
The preceding properties are generic to most resource types. After setting those values, you need to set the properties that are specific to the resource type you’re deploying.
Use intelligence or template reference to determine which properties are available and which ones are required. The following example sets the remaining properties for a public IP address,
Define variable
When defining a variable, you don’t specify a data type for the variable. Instead, provide a value or template expression. The variable type is inferred from the resolved value. The following example sets a variable to a string.
String Interpolation
Bicep templates allow using string interpolation. You can define strings using a parameter or variable name, and Bicep will evaluate the final string using the actual values.
Define output values
The following example shows how to use the output keyword to return property from a deployed resource.
In the following example, pip is the identifier (symbolic name) of a public IP address deployed in the Bicep file. The output value gets the fully qualified domain name for the public IP address.
Define modules
Every Bicep file can be consumed as a module. A module only exposes parameters and outputs as contracts to other Bicep files. Both parameters and outputs are optional.
The following Bicep file can be deployed directly to create a storage account or be used as a module.
Reference
https://github.com/ashwini19101994/azurebicepVMcreation.git
Visualize resources
You can view a representation of the resources in your file. From the upper left corner, select the visualizer button to open the Bicep Visualizer. The visualizer shows the resources defined in the Bicep file with the resource dependency information.
Deploying Bicep Templates
Now that you have a Bicep template built, it is time to deploy! You have two options for deploying Bicep templates. You can deploy using the Bicep template or convert the template to ARM JSON for deployment.
To deploy using the Azure CLI, use the az deployment group create command specifying the resource group name and template file.
az deployment group create\
–resource-group AzureBicep-RG\
–template-file vmcode.bicep
Overview of resources from azure portal
Clean up resources
az group delete –name AzureBicep-RG
Closing Note
Yes! Azure Bicep is a modern language and taking place in the market with its simplicity.
- Visual Studio Code– If you don’t already have Visual Studio Code, install it.

To verify you’ve installed the extension, open any file with the. bicep file extension. You should see the language model in the lower right corner change to Bicep.








You can specify minimum and maximum lengths for string and array parameters. You can set one or both constraints. For strings, the length indicates the number of characters. For arrays, the length indicates the number of items in the array.
The following example declares a parameter that must have 5 characters.



You can use expressions with the default value. You can’t use the reference function or any of the list functions in the parameters section. These functions get the resource’s runtime state and can’t be executed before deployment when parameters are resolved.






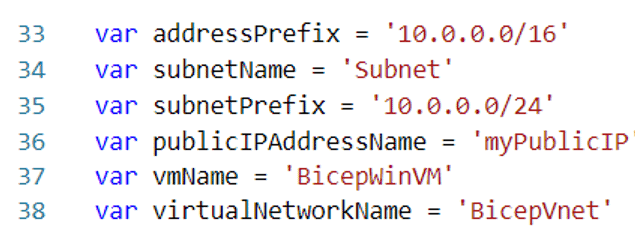




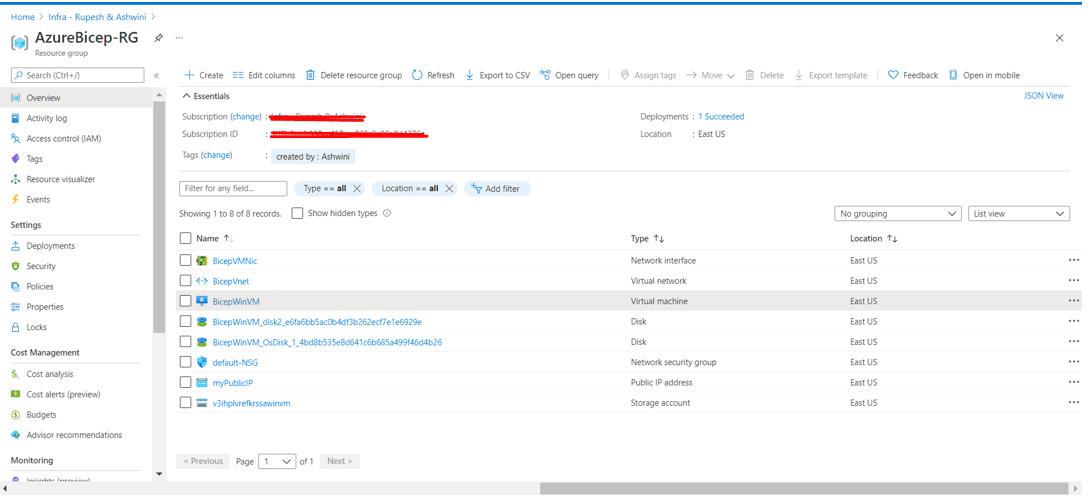
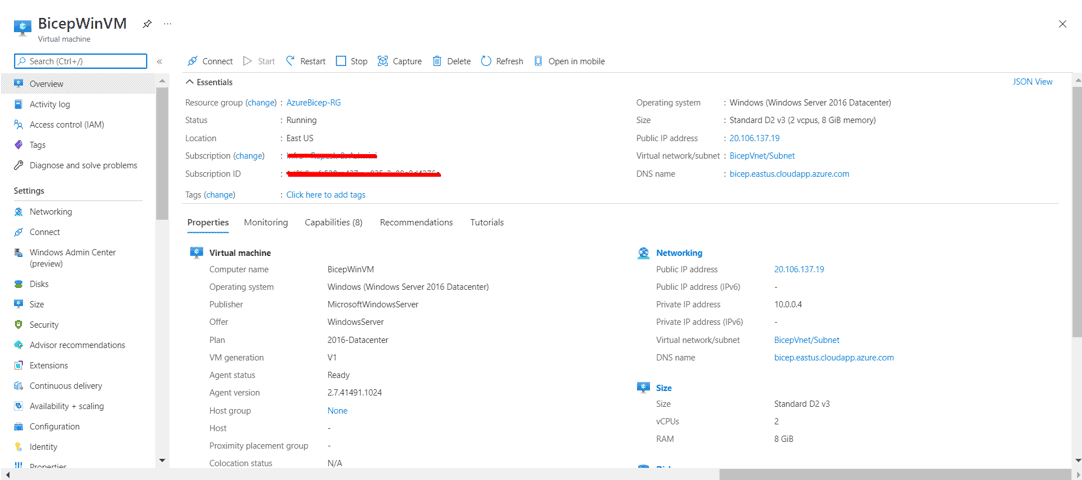